方案一:利用系统的CoreImage
(滤镜)
重点理解CIImage
,CIFilter
,CIContext
,CGImageRef
滤镜处理的过程比较慢,会造成加载图片缓慢的现象(等一会才看到图片),尽量放到子线程执行
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45
| - (void)viewDidLoad { [super viewDidLoad];
UIImage *image = [UIImage imageNamed:@"che"]; CIImage *ciImage = [[CIImage alloc] initWithImage:image]; CIFilter *blurFilter = [CIFilter filterWithName:@"CIGaussianBlur"]; [blurFilter setValue:ciImage forKey:kCIInputImageKey]; NSLog(@"%@", [blurFilter attributes]); [blurFilter setValue:@(2) forKey:@"inputRadius"];
CIImage *outCiImage = [blurFilter valueForKey:kCIOutputImageKey];
CIContext *context = [CIContext contextWithOptions:nil]; CGImageRef outCGImage = [context createCGImage:outCiImage fromRect:[outCiImage extent]]; UIImage *blurImage = [UIImage imageWithCGImage:outCGImage]; CGImageRelease(outCGImage); UIImageView *imageV = [[UIImageView alloc] initWithFrame:CGRectMake(0, 0, 750 / 2, 1334 / 2)]; imageV.image = blurImage; imageV.center = self.view.center; [self.view addSubview:imageV]; }
|
方案二:利用UIImage+ImageEffects分类
- 将
UIImage+ImageEffects.h
和UIImage+ImageEffects.m
文件加载进工程
- 包含
UIImage+ImageEffects.h
UIImage+ImageEffects
文件路径
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| #import "ViewController.h"
#import "UIImage+ImageEffects.h" - (void)viewDidLoad { [super viewDidLoad];
UIImage *sourceImage = [UIImage imageNamed:@"che"];
UIImage *blurImage = [sourceImage blurImageWithRadius:10]; UIImageView *imageV = [[UIImageView alloc] initWithImage:blurImage]; [self.view addSubview:imageV];
}
|
方案三:利用UIVisualEffectView(iOS8)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56
| #import "ViewController.h"
@interface ViewController ()
@property (nonatomic, strong) UIScrollView *scrollView;
@end
@implementation ViewController
- (void)viewDidLoad { [super viewDidLoad];
self.scrollView = [[UIScrollView alloc] initWithFrame:self.view.bounds]; UIImageView *imageV = [[UIImageView alloc] initWithImage:[UIImage imageNamed:@"fengjing"]]; self.scrollView.contentSize = imageV.image.size; self.scrollView.bounces = NO; [self.scrollView addSubview:imageV]; [self.view addSubview:self.scrollView]; UIVisualEffectView *effectView = [[UIVisualEffectView alloc] initWithEffect:[UIBlurEffect effectWithStyle:UIBlurEffectStyleLight]]; effectView.frame = CGRectMake(0, 100, 375, 200); [self.view addSubview:effectView]; UILabel *label = [[UILabel alloc] initWithFrame:effectView.bounds]; label.text = @"模糊效果"; label.font = [UIFont systemFontOfSize:40]; label.textAlignment = NSTextAlignmentCenter; UIVisualEffectView *subEffectView = [[UIVisualEffectView alloc] initWithEffect:[UIVibrancyEffect effectForBlurEffect:(UIBlurEffect *)effectView.effect]]; subEffectView.frame = effectView.bounds; [effectView.contentView addSubview:subEffectView]; [subEffectView.contentView addSubview:label]; }
@end
|
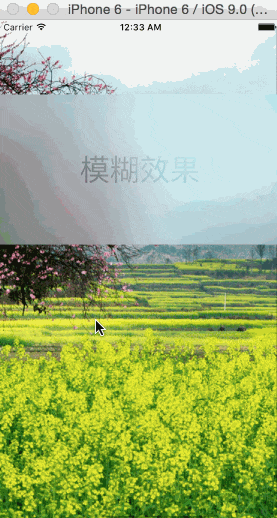